Lab 3 - Web security
In this lab we will be focusing on analysing the security of web applications.
Web applications are extremely vulnerable in the sense that they mix a multitude of technologies and libraries and feature highly dynamic pages and content that are often difficult to enclose and characterise.
Instead of focusing on exploitation, we will study how various existing analysis tools can help developers in detecting and fixing web vulnerabilities:
- SAST: As for low-level C programs, various vulnerability scanners such as SonarCloud and LGTM also have good support for analysis the source code of web applications written in various languages (HTTP/PHP/JS/TS/Java/etc).
- DAST: In particular for web applications, vulnerability assessment tools such as BurpSuite Community Edition and OWASP Zed Attack Proxy can be used to navigate web sites, analyse HTTP requests/responses and perform automatic dynamic scans for vulnerabilities.
There exists a myriad of vulnerable web applications, e.g. from the OWASP Vulnerable Web Applications Directory (VWAD), that have been developed for demonstrating common web vulnerabilities and how to exploit them.
One of the most modern and complete among such pedagogical web applications is the OWASP Juice Shop.
Another good resource with detailed information about each type of vulnerability, including how to manually test if your application is vulnerable, is the OWASP Web Security Testing Guide.
Lab install
To install the specific tools that will be used in this lab, run:
cd ses/vm
git pull
sh install-web.sh
In this lab we will be looking into solving a few Juice Shop challenges. You will find a lot of information in the Juice Shop companion guide.
Solving a challenge requires a basic understanding of an exploitation technique and crafting careful payloads that can get the attack done. In such a setting, reading previous solutions or looking at the project’s source code is considered sort of cheating. For this lab, the focus is on analysing the vulnerabilities; we encourage you to search the many available additional resources for solving the challenges as you see fit (while trying not to take all the fun out of it).
Interesting for us, many Juice Shop challenges also feature:
- a mitigation link with pointers to the OWASP cheat sheet related to the vulnerability;
- a coding challenge that will open a dialog containing the actual code snippet responsible for the security vulnerability behind the challenge and ask the challenger to understand the code and suggest the best fix.
There are various options to deploy an instance of Juice Shop, all listed in the GitHub page.
For a quick start, you may create a Docker container. However, for some tasks (and maximum vulnerability!) you will need to install from sources.
Inside the vm folder, you may just type:
make run-docker-juiceshop
to build and run from a Docker container. An instance of Juice Shop will be readily listening at http://localhost:3000
.
make build-juiceshop
to build from sources.
make run-juiceshop
to run from built sources. An instance of Juice Shop will be readily listening at http://localhost:3000
.
Threat Modelling
In the theoretical classes you have already studied the importance of threat modelling for understanding the security of a system.
More details
The Juice Shop developers have defined a general threat model [here](https://github.com/juice-shop/juice-shop/blob/master/threat-model.json), that provides an overview of the Juice Shop architecture, depicted below.
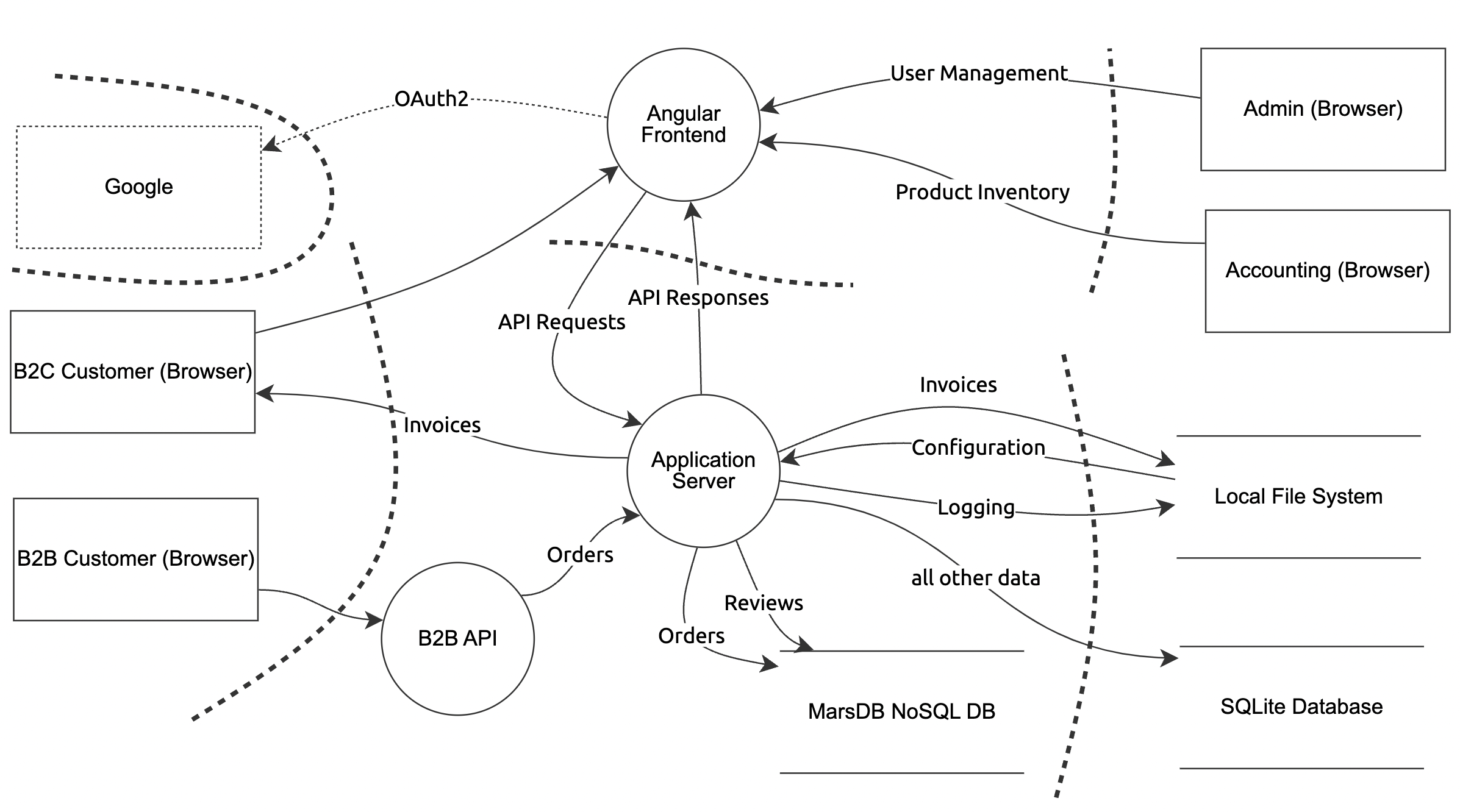
The model was created with the [OWASP Threat Dragon](https://owasp.org/www-project-threat-dragon/) tool. You may load the JSON file into the [online tool](https://www.threatdragon.com/) to visualize or edit the model.
Dependency scanning
In a real software development life cycle, scanning for vulnerabilities within external libraries is a natural starting point. THis is not only useful information while choosing the right dependencies for your project, but also important during the development and testing of your applications. It wouldn’t be very surprising to find a few of them in Juice Shop…
More details
### [Snyk](https://snyk.io/)
Snyk is free-to-use comercial tool that can automatically search the dependencies of a project for known vulnerabilities.
To run it for Juice Shop, do the following inside the [vm](../vm) folder:
```ShellSession
$ make build-juiceshop #you may skip if already build
$ cd juice-shop
$ snyk auth # this will require you to authenticate with the snyk online service
$ snyk test --json | snyk-to-html -o report.html
```
How many dependency vulnerabilities will you find for Juice Shop? You may look at a pre-generated report [here](https://hpacheco.github.io/ses/labs/lab3/snyk_report.html).
### [OWASP Dependency Check](https://owasp.org/www-project-dependency-check/)
Dependency-Check is a OWASP tool that attempts to detect publicly disclosed vulnerabilities contained within a project’s dependencies. Although it may feature a higher rate of false positives, it provides a more detailed report linking to the associated CVE entries.
In order to run Dependency-Check for Juice Shop, `cd` into your [vm][../vm] folder and run:
```Shell
make run-dependency-check
```
A pre-generated report for Juice Shop may be found [here](https://hpacheco.github.io/ses/labs/lab3/dependency-check-report.html).
Server scanning
Before we look at specific web vulnerabilities, let’s first make a preliminary recognition of the structure of our web page.
This is also useful for completing the JuiceShop score board challenge, which asks us to find a hidden web page which provides information about available challenges and keeps track of our challenge solving status.
More details
### [nikto](https://cirt.net/Nikto2)
Nikto is a web server scanner which performs comprehensive tests against web servers for multiple items, including potentially dangerous files/programs and server configuration or version-specific problems. Nikto is also highly configurable. We can run a basic nikto scan on our web page as follows and obtain a HTML report:
```Shell
nikto -o report.html -h http://localhost:3000
```
You may check a previously-generated report for OWASP Juice Shop [here](https://hpacheco.github.io/ses/labs/lab3/nikto.html).
### [Browser development toolkits](https://pwning.owasp-juice.shop/part1/rules.html)
As you navigate through a web site, the Javascript console, the site debugger and the HTTP requests are a valuable source of information.
In Firefox, you can open the Development Panel by clicking `F12`.
Hints for finding the hidden score board:
* you may search all files in the current web page with (`CTRL+SHIFT+F` in Firefox with all files closed).
* you can also easily dump the content of a web page to your computer and then search locally, e.g., to recursively download a web page with wget just try:
```Shell
wget -r http://localhost:3000
```
Dynamic Application Security Testing (DAST)
In the context of web applications, DAST is a testing methodology that analyses a running application by scanning and performing attacks on the client-side frontend. Since it is independent of the technologies used in the application’s server-side backend, it is also termed as a black-box testing methodology.
ZAP is an open-source OWASP-supported DAST tool for web applications.
ZAP provides automated scanners as well as a set of tools that allow you to find security vulnerabilities manually.
For further reference consult the online documentation.
More details
ZAP essentially supports the following modes:
* *Passive Mode*, where it simply works as a proxy that intercepts all requests and analyzes them for finding vulnerabilites;
* *Active Mode*, where it actively crawls the visited web pages to search for new vulnerabilities.
Naturally, you can always combine both modes for maximum coverage.
For instance, start by running an *Automated Scan* on http://localhost:3000.
However, a common hurdle for web scanners is that any vulnerability in a page that requires authentication will not be found by the *Automated Scan*, since ZAP will not be able to login into the page.
You may overcome this limitation by running a *Manual Explore* on http://localhost:3000.
It will launch a new browser instance proxied via ZAP network traffic monitors.
Turn on the *Attack Mode* and login into one of the user accounts; you may navigate through the site and run a traditional *Passive Spider* analysis to detect web pages; then run an *Active Scan* to detect further vulnerabilities.
For the case of Juice Shop, an interesting detail is that it comes with a large set of end-to-end (E2E) tests. The main purpose of E2E testing is to test the end user's experience by simulation real scenarios using the web application's interface. For the case of Juice Shop, E2E tests attempt to exploit the web page to solve each challenge. Juice Shop's E2E tests are written using [Selenium](https://www.selenium.dev/) scripts to emulate user interactions with the browser.
So, a nice idea (originally from this [blog post](https://www.omerlh.info/2018/12/23/hacking-juice-shop-the-devsecops-way/)) to maximize vulnerability coverage would be to include these tests in ZAP scan to automate an otherwise manual search for known vulnerabilities. It turns out that the only thing that we need to do is compile Juice Shop from sources and proxy the E2E tests via ZAP. Since ZAP scans are likely to run for a really long time, a pre-generated report for Juice Shop, covering the E2E tests followed by an *Active Scan* can be found [here](https://hpacheco.github.io/ses/labs/lab3/2022-02-22-ZAP-Report-e2e-active.html).
API Testing
A crucial element of a web application is its API, which mediates communication between the backend and the frontend.
By nature, APIs expose application logic and sensitive information and are a prime target for attackers.
As an example, the OWASP API Security project highlights security risks and mitigation strategies for developing secure APIs. For the case of Juice Shop, you may find a mapping between challenges and API Security categories in the guide.
More details
The [OpenAPI Specification](https://swagger.io/specification/) defines a standard meta-language for describing the interface of RESTful APIs, which also facilitates the study of their security. There is a large ecosystem of tools to generate server/client code from API specifications of vice-versa infer API specifications from existing application code.
A natural direction and an important asset during the development of a secure web application is therefore to able to test an API against its specification. Various DAST tools support OpenAPI specifications, e.g., [ZAP](https://www.zaproxy.org/docs/desktop/addons/openapi-support/) can crawl an API specification.
For Juice Shop, we can test two existing OpenAPI specifications:
* a complete specification of the hidden API for B2B orders, available [here](https://github.com/juice-shop/juice-shop/blob/master/swagger.yml);
* an incomplete specification of a small subset of the Juice Shop client API, found [here](https://github.com/apox64/RestSec/blob/master/restsec-samples/src/main/resources/docs_swagger/swagger-juiceshop.json).
You can inspect them using the online [Swagger Editor](https://editor.swagger.io/).
*The following API testing tools will not be very useful for finding Juice Shop vulnerabilities. Since (1) it is easier to generate code from the API specification than infer a specification from the API code and (2) the quality of the testing depends very much on the preciseness and completeness of the API specification, they are most effective if adopted from the start. They are presented below because they may prove to be valuable tools to integrate the design and implementation processes of your project.*
#### [Schemathesis](https://github.com/schemathesis/schemathesis)
Schemathesis is a tool that uses property-based testing for checking the conformance of an implemented API against a specification.
Property-based testing is a more lightweight fuzzing-like technique that consists of generating random inputs - in this case API requests - oriented to the testing of a specific output property - in this case conformance of API responses w.r.t. an OpenAPI specification.
In fact, a great deal of the Juice Shop vulnerabilities that we will explore in the challenges below are related to care-free API implementations: accepting more request parameters than expected, retrieving more response parameters than expected, leaking internal state alongside non-gracefully-handled error messages, etc.
You can quickly try out schemathesis as follows; make sure that Juice Shop is running. In the [vm](../vm) folder:
```ShellSession
$ git pull
$ sh install-schemathesis.sh
$ cd juice-shop
$ schemathesis run swagger-juiceshop.json --checks all --base-url http://localhost:3000
```
The tool will try to generate various random requests that satisfy the request schema, and check if it receives 5xx server errors or responses that do not satisfy the response schema. The output may vary (depending on the randomness) but you shall see some failing tests.
OpenAPI also supports the specification of necessary security, such as authentication, that should be required to perform certain operations.
For Juice Shop, that is the case of the B2B order interface. Log in into the Juice Shop in your browser, and inspected requests/responses for the header `Authorization: Bearer `. You can then test the B2B interface with your secret `` as follows:
```ShellSession
$ schemathesis run swagger.yml -H "Authorization: Bearer " --checks all --base-url http://localhost:3000
```
By default, schemathesis tests each API operation independently. In real applications, API operations may have dependencies, e.g. getting the details of a product may only work for existing products, which may have been listed before.
In order to improve the precision of the generated tests, schemathesis also supports [stateful testing](https://schemathesis.readthedocs.io/en/stable/stateful.html) by using [OpenAPI links](https://swagger.io/docs/specification/links/), a new feature for describing how the values returned by one operation may be used as input for other operations.
#### [RESTler](https://github.com/microsoft/restler-fuzzer)
RESTler is a API fuzzing tool that searches for 5xx server errors. More interestingly, it also runs additional [stateful checkers](https://github.com/microsoft/restler-fuzzer/blob/main/docs/user-guide/Checkers.md) that search for fixed vulnerabilities in fuzz-generated sequences of operations that may have security implications, such as internal side-effects left by erroneous operations.
In the [vm](../vm) folder, run:
```ShellSession
$ git pull
$ sh install-restler.sh
$ cd juice-shop
```
RESTler works in 4 steps; for our Juice Shop client API:
1. **Compile** the specification
```ShellSession
$ Restler compile --api_spec swagger-juiceshop.json
```
2. **Test** the coverage of the specification
```ShellSession
$ Restler test --grammar_file Compile/grammar.py --dictionary_file Compile/dict.json --settings Compile/engine_settings.json --no_ssl
```
3. **Fuzz-lean**: quickly fuzz a few inputs per operation
```ShellSession
$ Restler fuzz-lean --grammar_file Compile/grammar.py --dictionary_file Compile/dict.json --settings Compile/engine_settings.json --no_ssl
```
4. **Fuzz**: long fuzz during a `time_budget` in hours
```ShellSession
$ Restler fuzz --grammar_file Compile/grammar.py --dictionary_file Compile/dict.json --settings Compile/engine_settings.json --no_ssl
```
You can find a more detailed description of these steps in the [tutorial](https://github.com/microsoft/restler-fuzzer/blob/main/docs/user-guide/TutorialDemoServer.md).
RESTler shall find a few simple errors with the Juice Shop client API.
For the B2B orders specification, you will need to obtain a valid JWT token, as before for Schemathesis.
Create a new script `token.sh` with executable permissions and the following content (the first line will be ignored):
```Shell
echo "{u'11111111-11111-1111-1111-1111111': {}}"
echo "Authorization: Bearer "
```
Repeat the 4 steps with the additional parameters `--token_refresh_interval --token_refresh_command token.sh`.
#### [Dredd](https://dredd.org/)
Dredd is another API testing tool with a focus on specification conformance.
In comparison with Schemathesis, Dredd is closer to unit testing and focuses more on complex test sequences and software development pipeline integration.
#### [Cherrybomb](https://github.com/blst-security/cherrybomb/)
Cherrybomb is a tool that permits checking OpenAPI specifications for good secure design practices. It also allows testing conformance of API w.r.t. their specification.
</details>
## Static Application Security Testing (SAST)
In the context of web applications, SAST is a testing methodology that analyzes source code to find security vulnerabilities.
In contrast to DAST, it only analyses components when they are at rest. Since it is language-depend and may inspect the code of both the server-side backend and the client-side frontend, it is also termed as a white-box testing methodology.
Automation and integration with the software development process are key considerations for SAST, in order to you want to continuously identify quality defects and security vulnerabilities as code is written.
The natural down-side of being a static analysis approach is that it is less precise, and may lead to a high number of false positives This is particularly true for web applications, that often integrate multiple programming languages, have few static compile-time guarantees and have complex behaviours and information flow.
### Source code vulnerability scanners
We have seen before that there exist various commercial and open-source SAST analysis tools that we may use to analyse source code in various language and integrate with our development. Most of these tools are particularly well-versed for analysing web applications.
When solving Juice Shop challenges, of course you can also cheat a bit and search the web page's [sources](https://github.com/juice-shop) for hints.
Even better, you may search for vulnerabilities using a SAST vulnerability scanner on the GitHub repository.
More details
This won't be necessary for the **Score Board** challenge, but keep the following pre-generated scans over Juice Shop as a reference for future tasks:
* [SonarCloud](https://sonarcloud.io/project/overview?id=hpacheco_juice-shop)
To scan your own code with these scanners, it is arguably more convenient to upload your code to a public git repository and run it through the online scanners. You may also fork the [juice-shop](https://github.com/juice-shop) git repository in order to test and analyse fixes to the `master` branch. Alternatively, you may try [GitLab](https://docs.gitlab.com/ee/user/application_security/secure_your_application.html), that offers free SAST support and paid DAST and API fuzzing services, or download the offline versions of [SonarQube](https://www.sonarqube.org/downloads/), [CodeQL](https://codeql.github.com/docs/codeql-for-visual-studio-code/analyzing-your-projects/) or [SemGrep](https://semgrep.dev/getting-started) and run them locally. You may also try [Coverity](https://scan.coverity.com/) online (login needed).
For the case of Juice Shop, we will be mainly interested in tools that can analyse JavaScript and TypeScript code.
Many generic code scanners also work for other languages. As an example, consider the following pre-generated scans over the PHP code of Mutillidae:
* [SonarCloud](https://sonarcloud.io/summary/overall?id=hpacheco_mutillidae)
There are also many other static web application analysis tools designed for specific languages languages. We name a few that you may try on your own for your projects:
* [Find Security Bugs](https://github.com/find-sec-bugs/find-sec-bugs/) is a static code analysis tool for Java web applications.
* [Enlightn](https://github.com/enlightn/enlightn) is a static security analyser for applications developed using the Laravel PHP framework.
* [Progpilot](https://github.com/designsecurity/progpilot) is a static taint analysis tool for PHP. It supports sanitizers, which allows more control over the taint analysis when compared to automated scanners such as SonarCloud.
* [Psalm](https://github.com/vimeo/psalm) is another security analysis tool for PHP. It supports user-controlled taint analysis much like Progpilot, but claims to perform better PHP type inference, which should reduce false positives significatively.
* [Python Taint](https://github.com/python-security/pyt) is a static analysis tool for Python web applications. Similar ideas have matured into the more recent Facebook [Pysa](https://developers.facebook.com/blog/post/2021/04/29/eli5-pysa-security-focused-analysis-tool-python/) project.
## Interactive Application Security Testing (IAST)
White-box dynamic web application analysis is sometimes also termed Interactive Application Security Testing (IAST).
Like traditional dynamic program analysis, IAST tools typically analyze running web applications by first instrumenting their code to better observe application behavior and data flow.
More details
Examples of open-source tools that we can classify as IAST are:
* GitLab [Jsfuzz](https://gitlab.com/gitlab-org/security-products/analyzers/fuzzers/jsfuzz) for fuzz testing of JavaScript code;
* Code Intelligence [jazzer.js](https://github.com/CodeIntelligenceTesting/jazzer.js) for fuzz testing of JavaScript code, that has integration with Google [OSS-Fuzz](https://google.github.io/oss-fuzz/getting-started/new-project-guide/javascript-lang/);
* [Gillian](https://gillianplatform.github.io/sphinx/js/symbolic-testing.html) for symbolic testing of JavaScript code.
*Unfortunately, IAST is very much dependent on used technologies since it requires significantly more integration with the host applications, are there are few open-source solutions (aside from hard to set up research prototypes) for us to experiment with. We will therefore focus only on DAST and SAST in our analysis of Juice Shop.*
## Challenges
### Improper input validation
One of the most common vulnerabilities in any software application relates to the potentially devastating impact that user input can have on the intended behavior of the application. For web applications, inputs are typically passed as arguments to HTTP requests.
In Juice Shop, there are various challenges related to [improper input validation](https://pwning.owasp-juice.shop/part2/improper-input-validation.html).
#### Zero Stars challenge
More details
Let's have a look at the **Zero Stars** challenge, which asks us to submit a review with a 0-star rating.
You may start by writing a review for any product, but surprisingly the review form has no associated rating.
However, in the Juice Shop's side menu you may notice the `Customer Feedback` page, available at `http://localhost:3000/#/contact`.
Try submitting a regular customer review and inspect the (legitimate) HTTP POST request that is sent to the server; unfortunately, the UI does not allow us to submit a 0-start rating.
Solution:
* [Basic] You may use the browser developer tools to find the HTTP request together with its arguments in JSON format.
You will notice that the request contains a `rating` field; setting that to `0` should do the trick!
We can concoct such an illegitimate request using a simple command line tool such as `wget` or `curl`.
* [Recommended] This simple attack is more convenient if we use a web monitoring tool such as OWASP ZAP or Burp Suite. If using ZAP, start a **Manual Explore** with url `http://localhost:3000/#/contact`. This will launch an instance of your browser extended with ZAP Proxy monitors, which will log all your HTTP requests. Submit a customer review, intercept the relevant request, and select the **Open/Resend with Request Editor** option to easily alter and resubmit your request.
#### Admin Registration challenge
More details
**Try to solve** the (very similar) **Admin Registration** challenge on your own.
After you have solved the challenge, follow the mitigation link to understand more about the associated vulnerability and solve the associated coding challenge.
### SQL injection
SQL injection (SQLi) is one of the most classical web attacks which may have devastating consequences on web applications suffering from associated vulnerabilities.
Juice Shop itself has a few challenges related to SQL injection vulnerabilities.
#### Login Admin challenge
More details
Let's start with the **Login Admin** challenge, which asks us login as an existing account with administrator privileges [^2].
If we navigate to `http://localhost:3000/#/login`, we can try to login by supplying an `email` (let's try `admin`) and a `password` (randomly `123`). We can also track the HTTP request sent to the server which shall look like:
```
POST http://localhost:3000/rest/user/login
{"email":"admin","password":"123"}
```
with response:
```OK
Invalid email and password.
```
[^2]: We can legitimately register a new user and login with it, and we can illegitimately make that user an administrator as we have seen before, but the goal here is to login with an existing administrator account without having its credentials.
Unsurprisingly, no luck so far. Now we need to start testing of the login fields are vulnerable to SQL injection.
A first attempt is usually to insert special characters in the request fields and see if that affects the response.
In SQL, strings are usually delimited with `'`.
So let's try sending a request with `email=admin'`. You shall get an error response with a `SQLITE_ERROR` (so we already know our backend DB is SQLite) and a description of the performed SQL query:
```SQL
SELECT * FROM Users WHERE email = 'admin'' AND password = '...' AND deletedAt IS NULL
```
This confirms our suspicion: a double enclosing delimiter in the email field leads to a syntax error.
We also learn something potentially dangerous about the query: the email selection comes before the match with the password, so can we comment out the password check? Yes we can, by sending a request:
```
POST http://localhost:3000/rest/user/login
{"email":"admin' --","password":"123"}
```
for which we get the response:
```OK
Invalid email and password.
```
Behind the scenes, the executed SQL query was:
```SQL
SELECT * FROM Users WHERE email = 'admin' -- AND password = '...' AND deletedAt IS NULL
```
We no longer get a syntax error since the query is valid. However, we do not get a valid login. This is because, by default, the server will log us as the first user that is returned by the selection; as it turns out that there is no user in the database with email `admin`, our selection is empty and we get a login error. You may check the source code for further clarification.
We can overcome this limitation easily, e.g., by returning all users in the `Users` database table, which is part of another challenge.
Let us inject SQL into the login field to bypass the login and login as the first user in the database, by sending the request:
```
POST http://localhost:3000/rest/user/login
{"email":"admin' OR TRUE --","password":"123"}
```
for which we get a successful login!
```
Behind the scenes, the executed SQL query was:
```SQL
SELECT * FROM Users WHERE email = 'admin' OR TRUE -- AND password = '698d51a19d8a121ce581499d7b701668' AND deletedAt IS NULL
```
Now the SQL query will check for `email = 'admin' OR TRUE` which is always `TRUE`, hence effectively selecting all users. If you check the `Account` in the Juice Shop, you will notice that you are logged in as `admin@juice-sh.op`; luckily for us, the administrator turned out to be the first user in the `Users` table.
##### Static taint analysis
The static code analysers will be able to find this vulnerability. The culprit will be in the file `routes/login.ts`.
if you inspect the logs, you will notice that some tools perform static taint analysis to be able to identify that the user input - origination from a POST request in the login form - may affect the SQL query.
##### [SQLmap](https://sqlmap.org/)
SQLMap is an open source penetration testing tool that automates the process of detecting and exploiting SQL injection flaws and taking over of database servers. Try running SQLMap over our login page as follows:
```Shell
sqlmap -u 'http://localhost:3000/rest/user/login' --data="email=*&password=*" --level 5 --risk 3 -f --banner --ignore-code 401 --dbms='sqlite'
```
The `--data` options tells SQLMap to send a POST request; `*` is treated as a wildcard standing for any string that SQLMap will try to fill. SQLMap shall find a vulnerable input similar to our previous successful exploit.
##### Fuzzing with OWASP ZAP
We can also use ZAP to automatically fuzz HTTP requests.
For detailed info, check the [wiki](https://www.zaproxy.org/docs/desktop/addons/fuzzer/) or this video [tutorial](https://www.youtube.com/watch?v=uSfGeyJKIVA).
After finding the user POST login form with a ZAP scan, select `Open/Resend with Request Editor`. Then select the email field and right-click `Fuzz`.
Next, you need to provide ZAP with a list of fuzz vectors, which are strings of common exploits to vulnerabilities [^3]. Conveniently, ZAP already comes with some lists. Select `Add Payload > File Fuzzers > jbrofuzz > SQL Injection`.
An `OK` response with an authentication token in its content demonstrates that the login form is vulnerable to SQLi. Note that, unlike with active or passive scanners, ZAP will not generate any alerts from fuzz testing; you always need to interpret the results manually.
[^3]: It is important to note that ZAP does not perform fuzzing in the traditional sense: it simply automates the process of testing a list of user-given inputs, and it will not try to mutate the inputs to find new inputs.
Screenshots
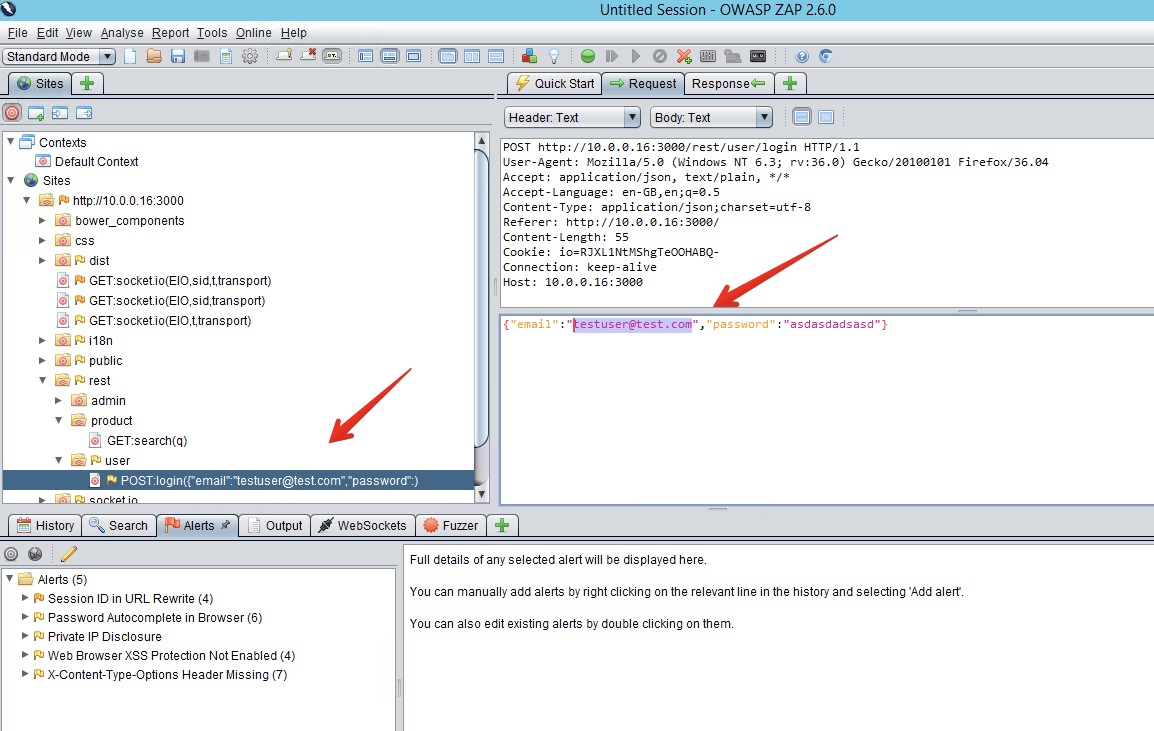
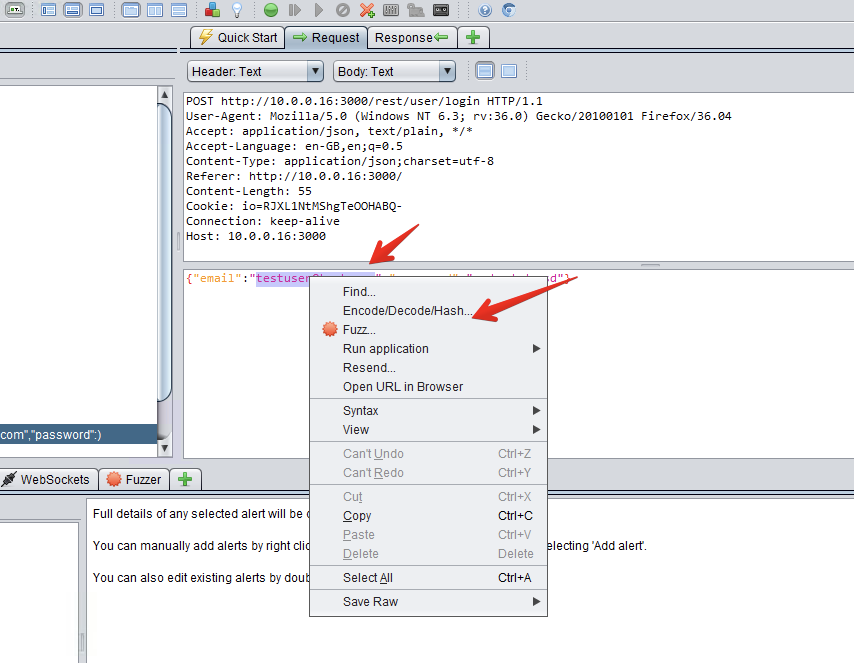
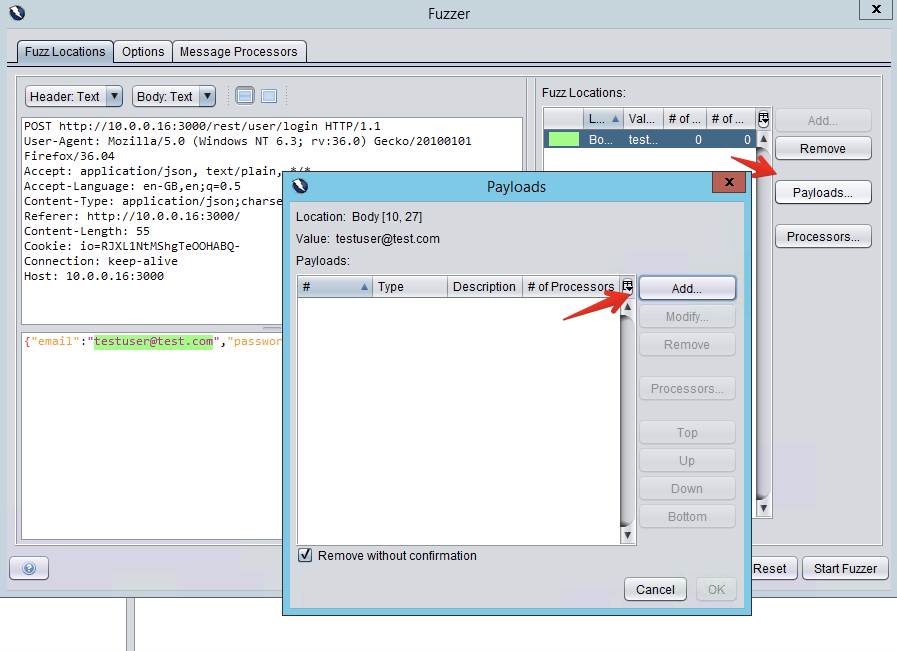
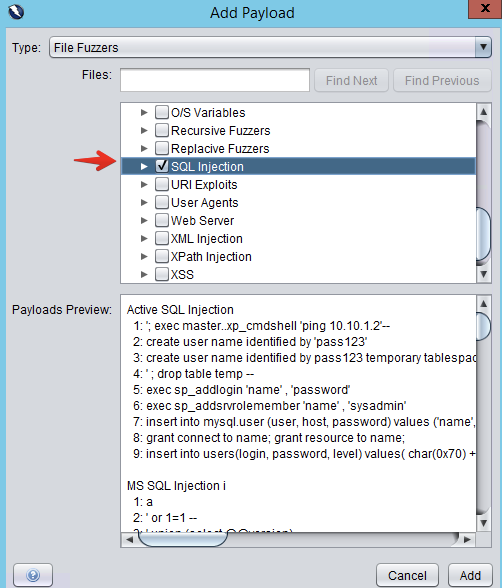
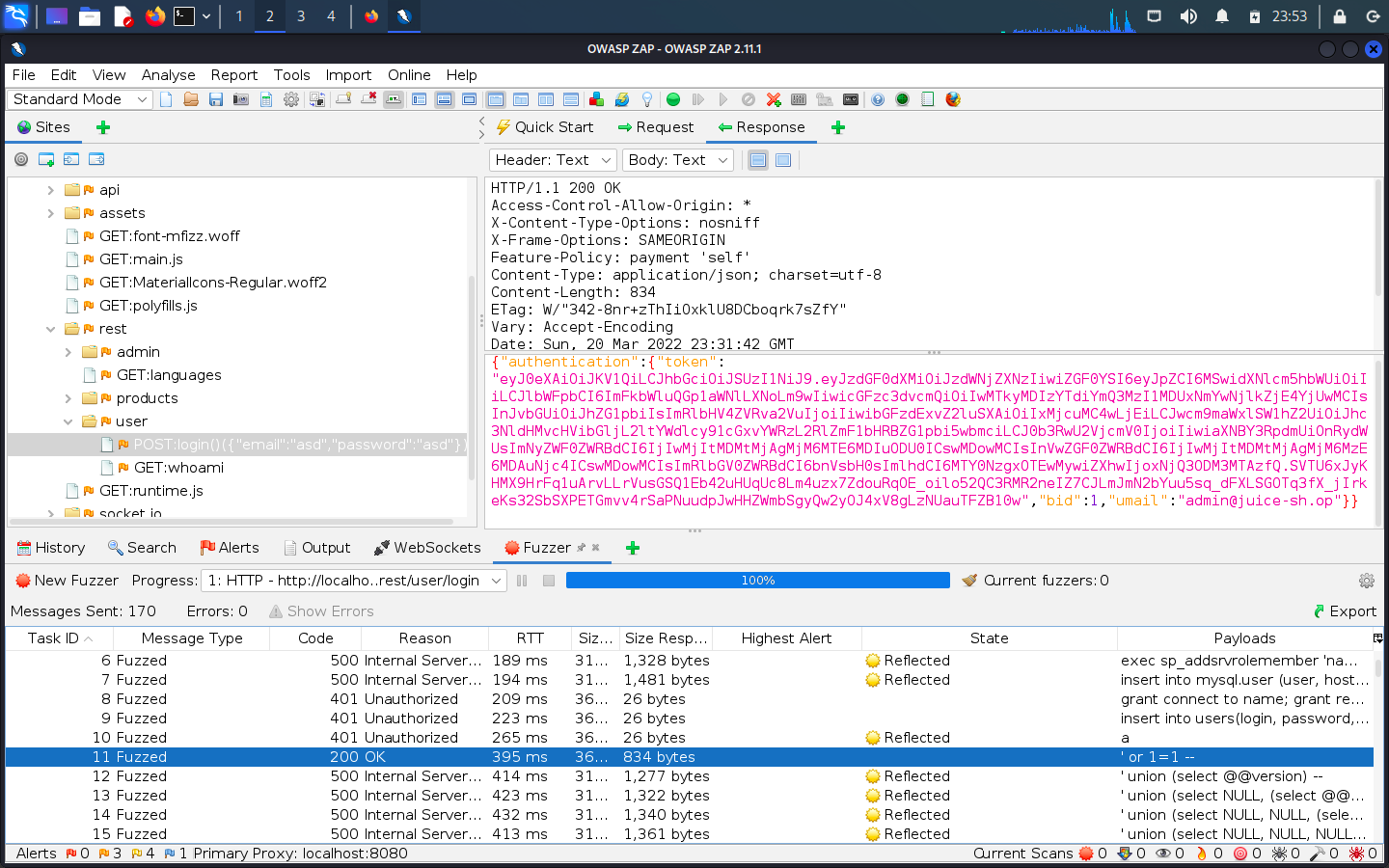
##### Fuzzing with [wfuzz](https://www.kali.org/tools/wfuzz/)
Wfuzz is a command-line alternative to ZAP, that comes pre-installed in Kali Linux.
It is also popular for other vulnerabilities beyond SQLi.
We can fuzz the user login POST request with the following command:
```Shell
wfuzz -c -z file,/usr/share/wordlists/wfuzz/Injections/SQL.txt -d “email=FUZZ&password=any” -u http://localhost:3000/rest/user/login
```
The `-c` flag makes color output.
The `-z` flag specifies a list of payloads (inputs) to the fuzzer.
The wfuzz tool will replace the keyword FUZZ with the words within the provided payload file; in this case, the `email` field of the POST data.
You will get an output similar to the shown below; successful logins can be distinguished form their `200 OK`responses.
Screenshots
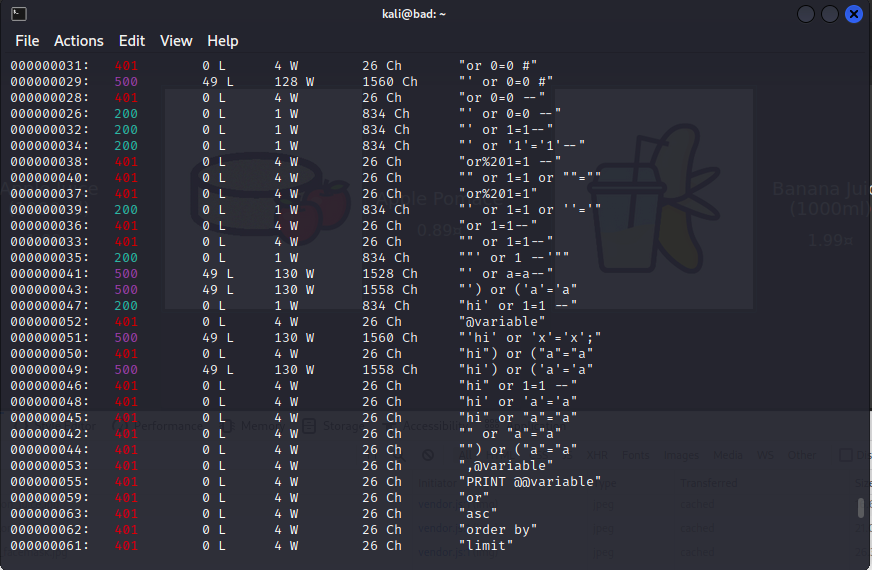
#### Other SQLi challenges
Juice Shop features a couple other SQL injection challenges.
**Try to solve by yourself two other challenges, one from each of the following pairs which are similar:**
* **Login Bender** or **Login Jim**: these challenges are very similar to the **Login Admin** and attack the login page, you just need to find the correct email first.
* **Database Schema** or **User Credentials**: these challenges require inferring some information about the database and attack the search page [^4].
After you have solved the challenges, follow the mitigation link to understand more about the associated vulnerability and solve the associated coding challenge.
[^4]: You shall be able to use SQLMap to easily assist you in leaking the whole database; explore the command-line options for more information on how to accomplish it. Note that Juice Shop may not be able to detect the SQLMap leak as a successful resolution of the challenge, because its detection mechanism is only looking out for certain queries.
##### GDPR Data Erasure challenge
More details
The **GDPR Data Erasure** is another SQLi-related challenge that requires combining the exploits of the above two classes.
Nonetheless, its origin goes beyond the SQLi vulnerabilities and can be attributed to a lack of proper GDPR compliance in the data erasure process.
**Try to solve it** by yourself and to trace back the problem to the [source code](https://github.com/juice-shop/juice-shop) responsible for the data erasure process. Can this problem be detected by the automated tools?
### Cross-Site Scripting (XSS)
XSS is another very common web vulnerability related to lack of input sanitization, which can take several forms:
* Stored XSS, a persistent form where a user can store malicious data in the database that will affect further requests;
* Reflected XSS, a non-persistent form where the user sends a malicious payload to the server which is not stored but instantaneously reflected back to the client;
* DOM-based XSS, a simpler form than a reflected XSS, where the attack takes place instantaneously in the client-side browser, without server intervention. From a user perspective, it is usually indistinguishable from a reflected XSS attack.
#### Dom XSS challenge
More details
Juice Shop features a multitude of XSS challenges, the simplest being the **Dom XSS** challenge.
In this challenge, we are asked to again exploit the search page, this time by inserting not malicious SQL code but malicious HTML code.
Let's start with some static and benign HTML: insert the search string `apple
` in the search form and check if your HTML code appears in the resulting page. If you inspect (using the browser's Developer mode) the resulting page's HTML source code, you shall easily find your inserted HTML code.
Looks good. Now let's try some JavaScript code: insert the search string `` in the search form in order to open a popup with message `XSS`.
This time, nothing happens... is the search field immune to XSS? Not at all, it just happens that our script is being inserted inside a `innerHTML` element and according to the [W3 HTML standard](https://www.w3.org/TR/2008/WD-html5-20080610/dom.html#innerhtml0):
> script elements inserted using innerHTML do not execute when they are inserted.
Check this [post](https://security.stackexchange.com/a/199850) for more details.
Luckily, there are many other XSS payloads that we can try. For example, our script will run if put inside an `iframe`; inserting the search string `